Welcome to our tutorial about change detection in Angular !
In this tutorial, we will learn how Angular’s change detection system works and how it can be used to build powerful and dynamic applications.
We will start by a little introduction to change detection in Angular. We will figure out what change detection is and why it is important.
Then We will also cover how to use the default change detection strategy in Angular and how to implement custom change detection strategies. This section will also cover when to use OnPush strategy.
By the end of this course, you will have a solid understanding of how Angular’s change detection system works and how to use it to build efficient and performant applications
Introduction to Change Detection in Angular
Change detection in Angular is a mechanism or a system that allows the framework to update automatically the view when the related data model changes.
This is a fundamental feature that allows Angular web applications to remain dynamic and responsive, without waiting for manual updates to the view.
In comparison to other JavaScript frameworks, Angular’s change detection system is more powerful and performant.
It uses a concept that allows it to skip the change detection on parts of the component view that are not concerned by the change.
This can be helpful to improve the performance of large and complex applications.
How The Change Detection is Working ?
In angular, the Change detection is implemented using a circular process that check the difference between two states (the current state and the previous state of the component)
Generally, The process starts by the root level component (root component) and then goes down through all the child components by checking for any changes.
If any change is detected, the view is updated to apply the new state.
General steps of the change detection process in Angular:
- Angular begins by executing the change detection operation on the root component.
- Angular runs change detection on all child components of the root component.
- Angular runs change detection on all child components of the child component, until all components and child component have been checked.
- If any change is detected by the change detector, Angular updates the view and apply the new state.
- Angular runs the same process to check for any new changes
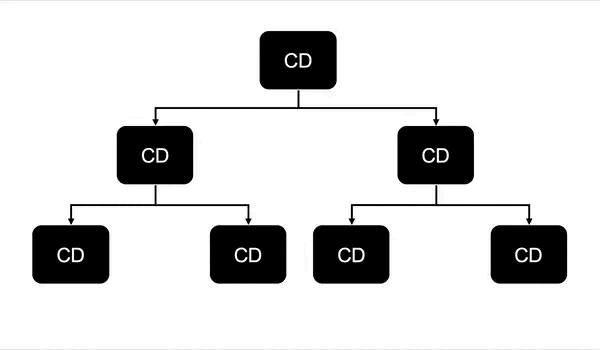
Change Detection Strategy in Angular
The change detection strategy in angular determines how the framework checks for changes in the data of the component and updates the related view.
Angular provides tow different strategies : Default strategy and OnPush strategy.
Default strategy
The default change detection strategy used in Angular is called “Default strategy”.
Using This strategy, angular will check for any changes on all components of the application for every detected event in the browser (button click, HTTP request, a timer…)
When we use the default change detection strategy, Angular will use a top-down approach, starting from a top-level component (root component) and then recursively checking all of its child components for changes.
If a change is detected in any component, Angular updates the related view
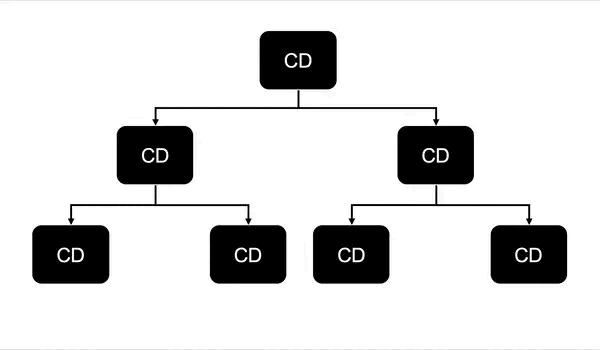
This strategy is very useful for small web applications, however it can be harmful for large applications because the change detector checks for every change in all the components on every event.
This can lead to slow down the performance of the application when we increase the number of the components.
Default strategy Example
This example show you haw to use the default change detection strategy in Angular :
Appcomponent.ts
file:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
{{counter}}
`
})
export class AppComponent {
counter = 0;
incrementCounter() {
this.counter++;
}
}
As you see in the code, we have created a method called incrementCounter()
that will be used to increment the property when we click on the button
Because we are using the default change detection strategy, when we click on the button to increment the value, the change detector will check for changes in all component including the current component.
OnPush strategy
The “OnPush” change detection strategy only checks for changes when an input property of the component changes or an event is emitted by the component.
Using this strategy can improve the performance of angular applications because the change detection will be run on the component only when it is necessary.
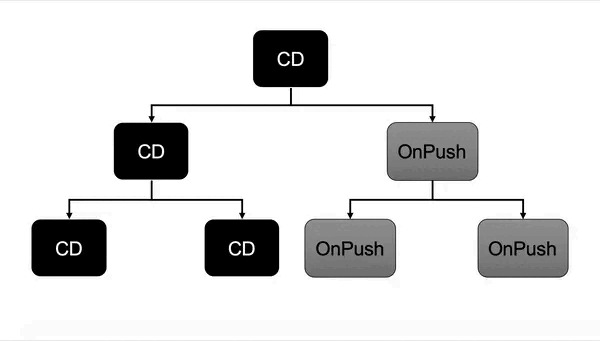
OnPush strategy Example
This example show you haw to use the “OnPush” change detection strategy in Angular :
Appcomponent.ts
file:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-root',
changeDetection: ChangeDetectionStrategy.OnPush,
template: `
{{counter}}
`
})
export class AppComponent {
counter = 0;
incrementCounter() {
this.counter++;
}
}
As you see in the code, we have used the same previous example, but we have changed the change detection strategy to see the difference.
We have set the onPush
strategy by adding the line (5) in to the @Component decorator
Using this change detection strategy, angular will check for changes in the current component only when the component input or any event emitted by the component.
In our case, when we click on the button, Angular will detect the change in the property “counter” and update the related view.