In this tutorial , we are going to see how to use ngAfterViewChecked lifecycle hook in angular and when angular exactly invokes the callback.
Also , we are going to see some differences between ngAfterViewChecked and ngAfterViewInit lifecycle hooks
Table of Contents
Angular Lifecycle Hooks
Angular has 8 lifecycle hooks, but in this tutorial, we will cover only the ngAfterViewChecked lifecycle hook
What is ngAfterViewChecked lifecycle hook ?
ngAfterViewChecked lifecycle hook is a callback which gets invoked by angular after the change detector completes the check of the directive or the child view.
ngAfterViewChecked lifecycle hook is invoked after ngAfterViewInit lifecycle hook and just before the last lifecycle hook ( ngOnDestroy ).
The ngAfterViewChecked lifecycle hook and ngAfterViewInit are useful when we use child components and directives.
So we can use the callback to add some custom functionalities after the change detector completes the check of the child component
But the important difference between ngAfterViewChecked and ngAfterViewInit is the number of times that angular invokes the callback.
ngAfterViewInit is called only one time when the component and child component are fully initialized,meanwhile ngAfterViewChecked lifecycle hook is called every time we make changes in parent component or the child component( button click … ).
That means , when we make changes in the parent or child component even if we don’t have any
@Inputs
, angular will invoke the ngDoCheck(1) of the parent then the ngDoCheck(2) of child components and that will automatically invokes the ngAfterViewChecked(3).
How to use the ngAfterViewChecked lifecycle hook ?
To correctly use the ngAfterViewChecked lifecycle hook and make a clean clode, we just need to implement the AfterViewChecked interface and use the callback method in the component like any other lifecycle hook.
Syntax :
@Component({selector: 'my-cmp', template: `...`})
class MyComponent implements AfterViewChecked {
ngAfterViewChecked() {
// ...
}
}
ngAfterViewChecked lifecycle hook Example
In this section , we are going to make a little example to check when angular invokes ngAfterViewChecked lifecycle hook.
In our example we are going to create a parent component and pass data to a child component using the property binding.
We are going to start by creating the parent component.
In the parent component template , we are going to inject the child component and update the property for every button click
to see when angular calls ngAfterViewChecked lifecycle hook.
It is a good opportunity to check also when calling ngAfterViewInit lifecycle hook and see the difference between them.
/* app.component.ts */
import {
AfterViewChecked,
AfterViewInit,
Component,
} from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements AfterViewChecked, AfterViewInit{
customDataFromParent = ''
setCustomDataToChild() {
let random = Math.floor(Math.random() * 10);
this.customDataFromParent = 'Custom data from Parent Component ' + random;
}
ngAfterViewChecked() {
console.log('ngAfterViewChecked was invoked from Parent')
}
ngAfterViewInit() {
console.log('ngAfterViewInit was invoked from Parent')
}
}
Parent Component
Now , we will create a child component which will be used by the parent to pass data using the property binding.
/*child.component.ts*/
import {Component, DoCheck, Input} from '@angular/core';
@Component({
selector: 'child-comp',
templateUrl: './child.component.html',
})
export class ChildComponent implements DoCheck {
@Input() customDataFromParent = '';
ngDoCheck() {
console.log('ngDoCheck was invoked from Child')
}
}
Child component
{{customDataFromParent}}
We will get something like this as a result :
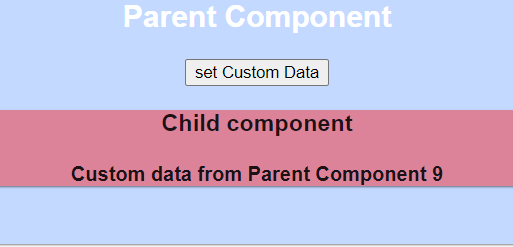
When we run the application , and see the logs inside the browser console , we will notice that the ngAfterViewChecked and ngAfterViewInit callbacks were invoked.
Now , when we click the button from the parent to update the property with a random number
we will notice that the ngAfterViewInit callback was not invoked and only the ngAfterViewChecked callback was invoked.
The example proves that the ngAfterViewChecked lifecycle hook is invoked every time we make changes in the view unlike the ngAfterViewInit lifecycle hook which is invoked only once.
References
Recapitulation
- ngAfterViewChecked lifecycle hook is useful when we use child components and directives
- ngAfterViewChecked is called every time we make changes in the parent or the child component
- ngAfterViewChecked is called just after the ngAfterViewInit when we load the component
- ngAfterViewChecked is called just after the ngDoCheck when we make changes
Clone The Project
To download and run the example, please follow the steps :
1- Clone the source code from XperTuto git repository using the following command :
git clone https://github.com/www-xperTuto-com/coming_soon.git
2- Install the node dependencies using NPM command line : npm install
3- Run the application using the following command : ng serve
4- Access to the project through the URL : http://localhost:4200
Read More About Angular Lifecycle hooks:
- How to use ngAfterViewInit lifecycle hook ?
- How to use ngOnChanges lifecycle hook ?
- How to use ngOnInit lifecycle hook ?
- How to use ngDoCheck lifecycle hook in angular ?
- How to use ngAfterContentInit lifecycle hook ?
- How to use ngAfterContentChecked lifecycle hook ?
- How to use ngAfterViewChecked lifecycle hook ?
- How to use ngOnDestroy lifecycle hook ?