In this tutorial , we are going to see how to use ngAfterContentInit lifecycle hook in angular and when angular exactly invokes the callback.
Also , we are going to see the difference between ngAfterContentInit and ngDoCheck lifecycle hooks through a simple example.
Table of Contents
Angular Lifecycle Hooks
Angular has 8 lifecycle hooks, but in this tutorial, we will cover only the ngAfterContentInit lifecycle hook
What is ngAfterContentInit lifecycle hook ?
As we know , angular provides 8 different lifecycle hooks to swiftly control the communication between components and especially the change detection system of our application.
In many cases , it is impossible to accomplish a task or fix an issue without using a specific lifecycle hook.
For example , it is nearly impossible to detect the changes on the @input() without using ngOnChanges or ngDoCheck lifecycle hook.
The ngAfterContentInit lifecycle hook is not used frequently as much as the other lifecycle hooks like ngOnInit , ngOnChanges or ngAfterViewinit , but there is always a purpose for using it.
The ngAfterContentInit callback invoked only once after three lifecycle hooks (ngOnChanges
, ngOnInit
and ngDoCheck
).
The ngAfterContentInit callback is called by angular after it has completed initialization of directive’s content .
So , if you are working with content projection (ng-content) , and you want to do something just after all the projected content has been initialized so you are in the right place , and you only need to use the ngAfterContentInit lifecycle hook to do the job.
How to use the ngAfterContentInit lifecycle hook ?
Generally , to know how to use the ngAfterContentInit lifecycle hook you need to know exactly when the callback is invoked by angular , otherwise your callback will never be invoked.
As we said in the previous section , the ngAfterContentInit lifecycle hook is useful if you are working with content projection and you need to do something just after all the projected content has been initialized
To correctly use the ngAfterContentInit lifecycle hook , we need to implement the AfterContentInit interface and use the ngAfterContentInit callback in the directive or the child component.
ngAfterContentInit lifecycle hook Example
In our example, we are going to create two components : parent component and child component ( the child component could be any directive)
We are going to start by creating the parent component.
In the parent component template , we are going to inject the child component with a custom content using the content projection concept.
We are going to update the projected content for every button click to see when exactly angular invokes the ngAfterContentInit lifecycle hook.
import {Component, } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements AfterContentInit {
customDataFromParent = ''
ngAfterContentInit() {
console.log('ngAfterContentInit was invoked')
}
setCustomDataToChild(): void {
let random = Math.floor(Math.random() * 10);
this.customDataFromParent = 'Custom data from Parent Component ' + random;
}
}
Parent Component
{{ customDataFromParent }}
Now , we are going to create the child component and use the to inject the projected content from the parent..
import {Component, DoCheck} from '@angular/core';
@Component({
selector: 'child-comp',
templateUrl: './child.component.html',
})
export class ChildComponent implements DoCheck {
ngDoCheck() {
console.log('ngDoCheck was invoked')
}
}
Child component
we are going to get something like this as a result :
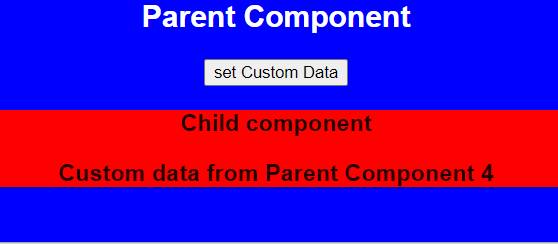
When we load the page , and see the logs , we will notice that the ngDoCheck and ngAfterContentInit callbacks were invoked.
Now , when we click the button from the parent to update the projected content with a random number ,
we will notice that the ngAfterContentInit callback was not invoked and only the ngDoCheck callback was invoked.
The example proves that the ngAfterContentInit lifecycle hook is invoked only once even if we update the projected content.
References
Recapitulation
In this tutorial we have seen how to use the ngAfterContentInit lifecycle hook in our project through a simple example , also we have seen how and when exactly angular invokes the AfterContentInit callback.
This tutorial helps us to see the difference between ngAfterContentInit and the other angular lifecycle hooks and that leads us to use the relevant lifecycle hook for each use case.
Brief :
- ngAfterContentInit is invoked only once after three lifecycle hooks
- ngAfterContentInit is called after it has completed initialization of directive’s content
- ngAfterContentInit is useful if you are working with content projection
- ngAfterContentInit is called just after all the projected content has been initialized
Clone The Project
To download and run the example, please follow the steps :
1- Clone the source code from XperTuto git repository using the following command :
git clone https://github.com/www-xperTuto-com/ngAfterContentInit_lifecycle.git
2- Install the node dependencies using NPM command line : npm install
3- Run the application using the following command : ng serve
4- Access to the project through the URL : http://localhost:4200
Read More About Angular Lifecycle hooks:
- How to use ngAfterViewInit lifecycle hook ?
- How to use ngOnChanges lifecycle hook ?
- How to use ngOnInit lifecycle hook ?
- How to use ngDoCheck lifecycle hook in angular ?
- How to use ngAfterContentInit lifecycle hook ?
- How to use ngAfterContentChecked lifecycle hook ?
- How to use ngAfterViewChecked lifecycle hook ?
- How to use ngOnDestroy lifecycle hook ?